Display ProgressBar in Android
Hey everyone!
A ProgressBar is basically a visual indicator of progress in some operation. Implementing a ProgressBar helps in the understanding of processes running on threads separate from the main User Interface (UI) and the communication between threads. As mentioned on the Android developer's page, a progress bar can also be made indeterminate. In indeterminate mode, the progress bar shows a cyclic animation without an indication of progress. This mode is used by applications when the length of the task is unknown.
By default the progress bar is a spinning wheel (an indeterminate indicator). We can always change to a horizontal progress bar using the Widget.ProgressBar.Horizontal style as follows,
<ProgressBar
style="@android:style/Widget.ProgressBar.Horizontal"
... />
Through this post, we will learn how to display a ProgressBar in Android.
Pre-requisites: Android SDK
Create a new Activity class called MyProgressBarActivity in any of your existing Android projects and add the following code!
MyProgressBarActivity.java
package com.example;
import android.os.Bundle;
import android.os.Handler;
import android.app.Activity;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
public class MyProgressBarActivity extends Activity {
private ProgressBar progBar;
private Handler mHandler = new Handler();;
private int mProgressStatus = 0;
private Button showProgressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
progBar= (ProgressBar)findViewById(R.id.progBar1);
showProgressBar= (Button)findViewById(R.id.btn1);
showProgressBar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
doSomeWork();
}
});
}
public void doSomeWork(){
// Start lengthy operation in a background thread
new Thread(new Runnable() {
public void run() {
while (mProgressStatus < 100) {
mProgressStatus += 1;
// Update the progress bar
mHandler.post(new Runnable() {
public void run() {
progBar.setProgress(mProgressStatus);
}
});
try {
//Display progress slowly
Thread.sleep(200);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
}
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/txt1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
<Button
android:id="@+id/btn1"
android:layout_below="@+id/txt1"
android:text="@string/show"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<ProgressBar
android:id="@+id/progBar1"
android:layout_below="@+id/btn1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
style="@android:style/Widget.ProgressBar.Horizontal"
android:layout_marginRight="15dp" />
</RelativeLayout>
Run the Android application by specifying the above Activity as the default launcher Activity and you should see the ProgressBar in action! :)
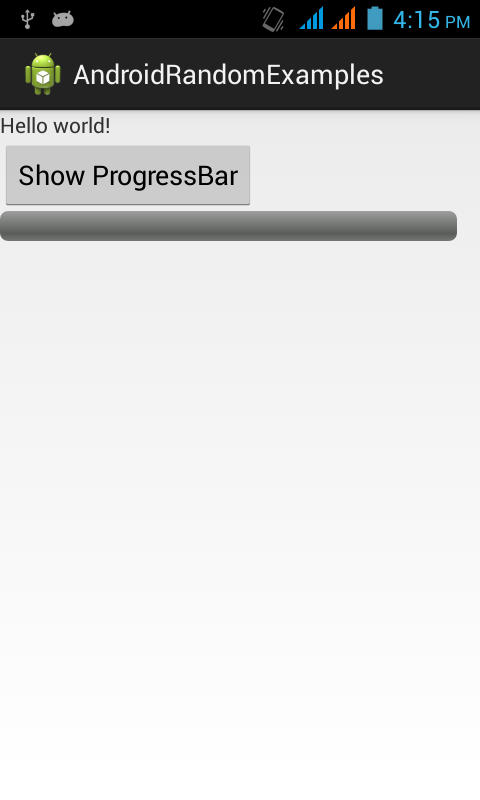
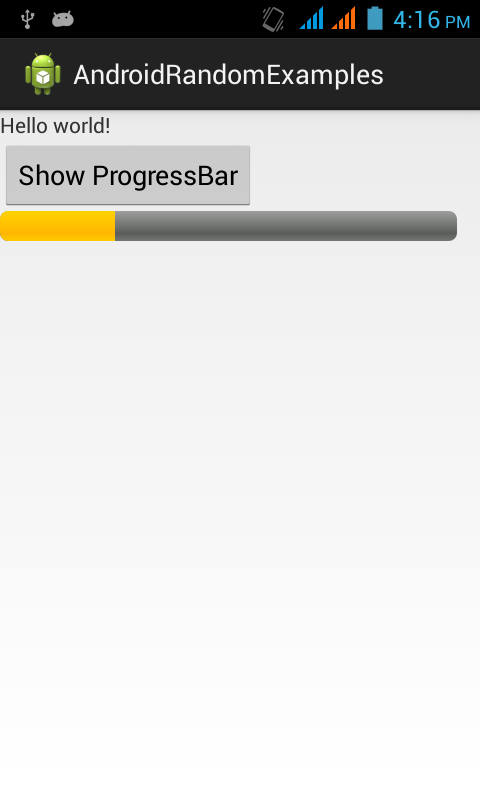